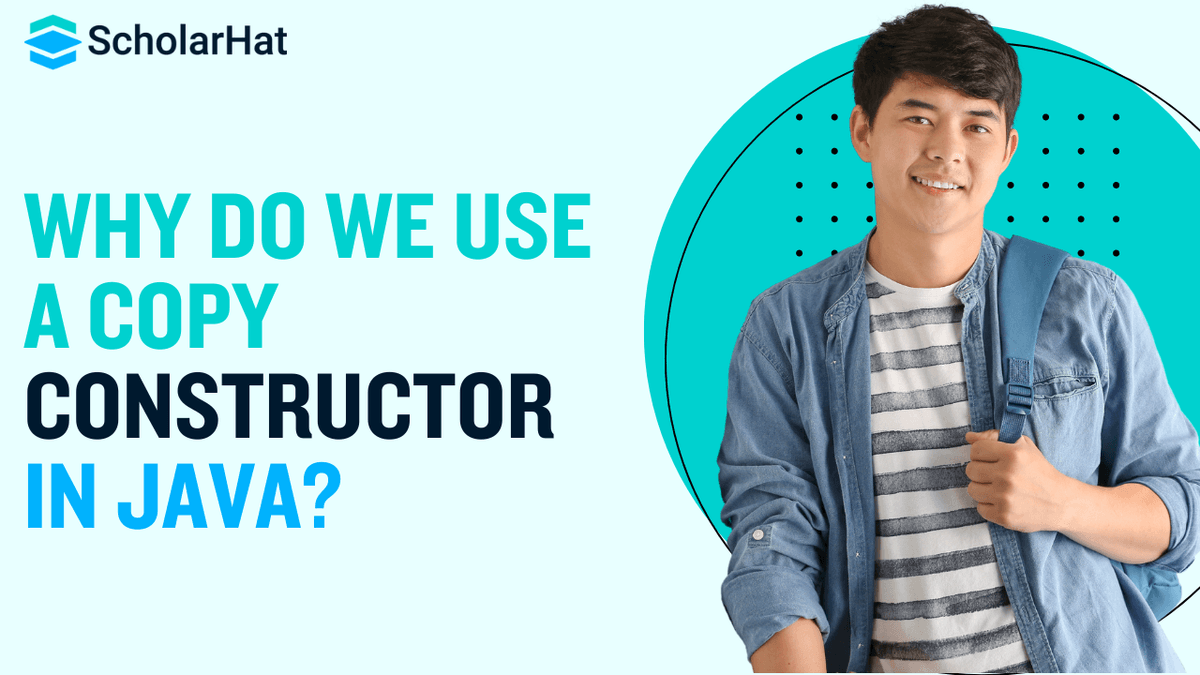
In Java programming, understanding the significance of a copy constructor in Java is crucial for effectively managing object-oriented principles and ensuring robust code structure. This article explores the purpose, implementation, benefits, and best practices associated with copy constructors, shedding light on their role in object creation and manipulation.
Introduction to Copy Constructor in Java
A copy constructor in Java serves the essential function of creating a new object as a copy of an existing object. It plays a pivotal role in object initialization and is particularly useful when you need to replicate an object's state without referencing the same memory location.
What is a Copy Constructor?
A copy constructor is a special constructor that takes an object of the same class as a parameter and initializes a new object using values from the existing object. It ensures that changes to one object do not affect the other, providing a mechanism for object cloning.
Purpose and Benefits of Using a Copy Constructor
1. Cloning Objects
The primary purpose of a copy constructor in Java is to clone objects. By creating a new instance with identical data to an existing object, it facilitates safe and independent manipulation of object states.
2.
Immutable Objects
In scenarios where immutability is desired, a copy constructor allows for the creation of immutable objects. Once initialized, the state of these objects cannot be changed, ensuring data integrity and thread safety.
3. Deep Copy vs. Shallow Copy
A copy constructor can be used to implement both shallow and deep copies of objects. A shallow copy duplicates the object's references, while a deep copy replicates the object along with all referenced objects recursively.
Implementation of Copy Constructor in Java
Syntax and Usage
public class MyClass {
private int value;
// Copy Constructor
public MyClass(MyClass original) {
this.value = original.value; // Copying data from original object
}
// Other constructors and methods
}
Example: Using a Copy Constructor
public class Main {
public static void main(String[] args) {
MyClass obj1 = new MyClass(10);
MyClass obj2 = new MyClass(obj1); // Using copy constructor to create obj2
}
}
Best Practices for Using Copy Constructors
1. Ensure Proper Initialization
Initialize all member variables in the copy constructor to maintain object consistency and prevent unintended behavior due to uninitialized state.
2. Handle Object References Carefully
When dealing with objects that contain references to other objects, ensure that the copy constructor correctly clones all referenced objects (deep copy) if necessary.
3. Documentation and Usage Guidelines
Document the usage and behavior of the copy constructor in your codebase to guide developers on when and how to use it effectively.
Comparing Copy Constructor with Constructor Overloading in Java
Constructor Overloading in Java
Constructor overloading in Java involves defining multiple constructors with different parameters within the same class. It allows for object initialization with varying sets of arguments, enhancing flexibility in object creation.
FAQs About Copy Constructor in Java
1. What is the difference between a copy constructor and a regular constructor in Java?
A copy constructor initializes a new object by copying the state of an existing object of the same class, while a regular constructor initializes a new object without reference to any existing object.
2. When should I use a copy constructor in Java?
Use a copy constructor when you need to create a new object that is a replica of an existing object, maintaining independence from the original object's state.
3. Can a copy constructor be used to perform deep copy of objects in Java?
Yes, a copy constructor can be implemented to perform a deep copy, ensuring that all referenced objects are also duplicated recursively.
4. Does Java provide a built-in copy constructor?
No, Java does not provide a built-in copy constructor. It must be explicitly defined in the class definition based on the specific requirements of object cloning.
5. What are the advantages of using a copy constructor over clone() method in Java?
A copy constructor offers simplicity and clarity in object cloning operations, whereas clone() method involves more intricate implementation and potential issues with object types and visibility.
6. Can a copy constructor throw exceptions in Java?
Yes, a copy constructor can throw exceptions if there are exceptional conditions during object cloning, such as null references or invalid state.
7. How do I test a copy constructor in Java?
Testing a copy constructor involves creating instances of the class, invoking the copy constructor with existing objects, and verifying that the new object's state matches the original object.
8. Is it mandatory to implement a copy constructor for every class in Java?
No, implementing a copy constructor is not mandatory for every class in Java. It is used selectively based on the need to clone objects and manage object states effectively.
9. What are the common pitfalls when using a copy constructor in Java?
Common pitfalls include incomplete copying of object state (shallow copy), forgetting to handle null references, and improper handling of object references during deep copy operations.
10. Can a copy constructor be private in Java?
Yes, a copy constructor can be made private in Java to restrict its accessibility and ensure controlled object cloning within the class or designated packages.
Conclusion
In conclusion, a copy constructor in Java serves as a powerful tool for object cloning, allowing developers to manage object states effectively and maintain data integrity. By understanding its purpose, implementation techniques, and best practices, you can leverage copy constructors to enhance the robustness and flexibility of your Java applications. For further exploration of copy constructors in Java, refer to this comprehensive guide on copy constructor in Java for detailed insights and practical examples.