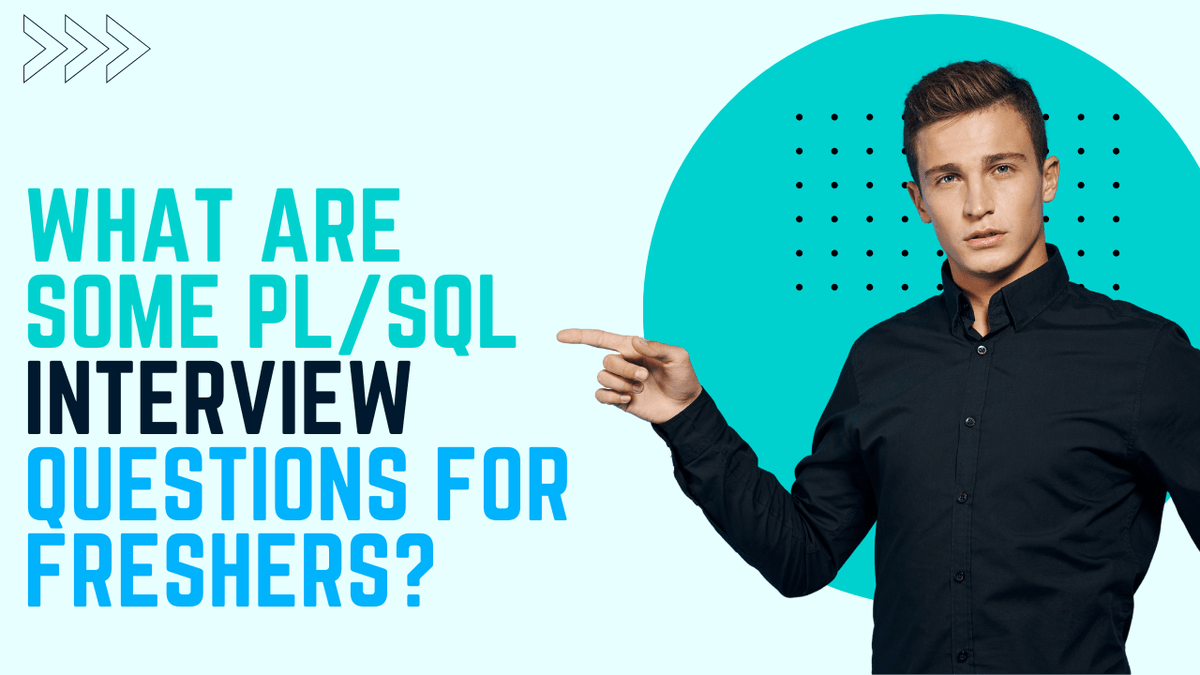
If you're gearing up for a PL/SQL interview, especially as a fresher, understanding the types of questions you might face is crucial. PL/SQL interview questions for freshers often test basic understanding, problem-solving skills, and knowledge of fundamental concepts. This article will guide you through common questions, providing insights and resources to help you prepare effectively. For those interested in other aspects of SQL, you can also explore various SQL interview questions for freshers.
Understanding PL/SQL Basics
Before diving into specific questions, it's essential to grasp the basics of PL/SQL. PL/SQL, or Procedural Language/Structured Query Language, is Oracle's procedural extension for SQL. It allows for more complex data manipulation and control through procedural constructs.
What Is PL/SQL?
PL/SQL is designed to seamlessly integrate with SQL and enhance its capabilities. While SQL is used to query and manipulate data, PL/SQL adds procedural features such as loops and conditional statements. This combination makes it a powerful tool for managing and automating database tasks.
Key Concepts to Know
- Blocks: PL/SQL code is organized into blocks, which can be anonymous or named. Understanding how to structure these blocks is fundamental for writing effective PL/SQL code.
- Variables: Variables in PL/SQL are used to store data temporarily during execution. Familiarity with declaring and using variables is critical.
- Control Structures: This includes conditional statements (IF-THEN-ELSE), loops (FOR, WHILE), and exception handling. Mastery of these constructs is often tested in interviews.
Common PL/SQL Interview Questions
1. What Is the Difference Between PL/SQL and SQL?
One of the PL/SQL interview questions for freshers might address the differences between PL/SQL and SQL. SQL is a standard language for querying and managing databases, while PL/SQL extends SQL with procedural capabilities. PL/SQL allows for the creation of complex logic and control structures, which SQL alone cannot handle.
2. Explain the Structure of a PL/SQL Block
This question tests your understanding of PL/SQL's structure. A PL/SQL block consists of three main sections:
- Declaration Section: Where variables, constants, and cursors are declared.
- Execution Section: Contains the executable statements. This is where the actual logic is implemented.
- Exception Handling Section: Handles any runtime errors that occur during execution.
3. What Are Cursors in PL/SQL?
Cursors are essential in PL/SQL for handling query results. There are two types:
- Implicit Cursors: Automatically created by Oracle for SELECT INTO statements.
- Explicit Cursors: Defined by the user for queries that return multiple rows. They offer more control over the context of the data retrieval.
4. How Do You Handle Exceptions in PL/SQL?
Exception handling is a critical aspect of PL/SQL. The EXCEPTION block is used to manage errors gracefully. Common exceptions include NO_DATA_FOUND, TOO_MANY_ROWS, and ZERO_DIVIDE. Understanding how to use exception handling helps ensure that your PL/SQL code is robust and error-resistant.
5. What Is a Stored Procedure in PL/SQL?
A stored procedure is a precompiled collection of SQL statements and PL/SQL blocks. It can be invoked to perform a specific task, such as data manipulation or validation. Stored procedures help in modularizing code and improving performance by reducing the need for repetitive code.
For a deeper dive into stored procedures, you can read more about different types of stored procedures in SQL.
6. How Do You Create and Use a Function in PL/SQL?
Functions in PL/SQL are similar to procedures but return a single value. They are useful for performing calculations or operations that produce a result. To create a function, you define it using the CREATE FUNCTION statement, specifying the return type and the logic within the function.
Practical Questions and Coding Challenges
7. Write a PL/SQL Block to Fetch Data Using a Cursor
Here's a typical question where you might need to write code on the spot. An example task could be to write a PL/SQL block that uses a cursor to fetch and display employee names from a table. Be prepared to demonstrate your ability to use cursors effectively.
8. How Would You Optimize a PL/SQL Program?
Optimization is crucial for performance. Discuss strategies like using bulk processing, minimizing context switches between PL/SQL and SQL, and avoiding unnecessary computations. Showing that you can write efficient and scalable code is key in an interview.
9. Demonstrate the Use of Collections in PL/SQL
Collections in PL/SQL include associative arrays, nested tables, and VARRAYs. A question might ask you to demonstrate how to declare and use these collections to manage and process multiple rows of data efficiently.
10. How Do You Implement Error Handling in PL/SQL?
Beyond basic exception handling, you might be asked to implement custom error handling. Discuss how to use user-defined exceptions and log errors for troubleshooting. Demonstrating a thorough approach to managing exceptions can set you apart.
Preparing for Your PL/SQL Interview
When preparing for PL/SQL interview questions, focus on both theoretical knowledge and practical skills. Practice writing PL/SQL code, understand key concepts, and be ready to explain your solutions clearly. Also, make sure to review other relevant topics such as database normalization, transactions, and SQL performance tuning.
Conclusion
In summary, preparing for PL/SQL interview questions for freshers involves understanding fundamental concepts, practicing coding, and being able to explain your approach to solving problems. By familiarizing yourself with these common questions and practicing your responses, you can boost your confidence and improve your chances of success in your upcoming interviews. For additional insights into SQL and its broader applications, you might find SQL interview questions for freshers useful. And don't forget to explore the intricacies of stored procedure in SQL to enhance your overall understanding.
FAQ
1. What is PL/SQL and why is it used?
PL/SQL, which stands for Procedural Language/Structured Query Language, is Oracle’s procedural extension to SQL. It combines SQL’s data manipulation capabilities with procedural constructs, such as loops and conditionals, to enable more complex and flexible data management and application logic. PL/SQL is used for writing scripts, stored procedures, and triggers in Oracle databases to automate tasks and ensure efficient data processing.
2. How does PL/SQL differ from SQL?
While SQL is used for querying and managing databases through statements like SELECT, INSERT, UPDATE, and DELETE, PL/SQL extends SQL with procedural programming features. This allows for the use of variables, loops, and exception handling, which are not available in standard SQL. PL/SQL is often used to create applications that require more complex logic beyond simple SQL queries.
3. What are the main components of a PL/SQL block?
A PL/SQL block consists of three main sections:
- Declaration Section: This is where you define variables, constants, cursors, and exceptions.
- Execution Section: This contains the executable statements that perform the main logic of the block.
- Exception Handling Section: This is used to catch and handle exceptions (errors) that occur during the execution of the block.
4. What is a cursor in PL/SQL and how is it used?
A cursor is a database object used to retrieve and manage query results. There are two types of cursors in PL/SQL:
- Implicit Cursors: Automatically created by Oracle for SQL statements that return a single row or a result set.
- Explicit Cursors: Defined by the user for queries that return multiple rows. They offer more control over row processing and allow for operations such as fetching, looping through rows, and closing the cursor.
5. How do you handle exceptions in PL/SQL?
Exception handling in PL/SQL is done using the EXCEPTION block, where you can catch predefined exceptions like NO_DATA_FOUND, TOO_MANY_ROWS, and ZERO_DIVIDE, or define your own custom exceptions. By handling exceptions, you can manage errors gracefully and ensure that your PL/SQL code continues to execute or terminates properly.
6. What is a stored procedure in PL/SQL?
A stored procedure is a precompiled collection of PL/SQL statements that perform a specific task. Stored procedures are stored in the database and can be invoked by applications or other PL/SQL blocks. They help in modularizing code, enhancing performance by reducing redundant SQL executions, and encapsulating business logic.
7. How can you optimize PL/SQL code for performance?
To optimize PL/SQL code, consider:
- Using Bulk Processing: Techniques like BULK COLLECT and FORALL can reduce context switches between PL/SQL and SQL.
- Minimizing Cursor Usage: Use cursors efficiently and close them properly to avoid resource leaks.
- Reducing Unnecessary Computations: Avoid redundant calculations and operations within loops.
8. What are PL/SQL collections and how are they used?
PL/SQL collections include:
- Associative Arrays: Index-by tables that allow for fast access using keys.
- Nested Tables: Tables that can store multiple rows, similar to a database table but used within PL/SQL.
- VARRAYs (Variable-Size Arrays): Arrays with a fixed upper bound on their size.
Collections are used to handle multiple rows of data in memory, providing efficient ways to store, retrieve, and manipulate sets of data.
9. How do you create and use a function in PL/SQL?
To create a function in PL/SQL, use the CREATE FUNCTION statement. Define the function with its name, parameters, return type, and body. The function can be used in SQL queries and other PL/SQL blocks to perform specific operations and return a single value. For example:
sql
Copy code
CREATE FUNCTION calculate_bonus (salary NUMBER) RETURN NUMBER IS
BEGIN
RETURN salary * 0.10;
END;
10. What should I focus on while preparing for a PL/SQL interview?
When preparing for a PL/SQL interview, focus on:
- Understanding Core Concepts: Make sure you understand the basics of PL/SQL, including blocks, variables, and control structures.
- Practicing Coding: Write and debug PL/SQL code to get comfortable with syntax and common scenarios.
- Exploring Practical Applications: Be prepared to discuss how you would handle real-world problems using PL/SQL.Reviewing Related Topics: Brush up on SQL concepts and other related topics to demonstrate a well-rounded knowledge base.